Web: ProcessOut.js
Integrate and customize Dynamic Checkout using our client-side Javascript SDK
ProcessOut.js enables the integration of Dynamic Checkout on Web, by importing our SDK hosted on our CDN. After creating your Dynamic Checkout configuration using our Dashboard or API you can enable the end-to-end Dynamic Checkout payment flow by importing the script on your website.
To set up the Dynamic Checkout Web SDK you will need to retrieve these parameters from your application’s backend:
- Project ID
- Invoice ID
- Client secret (optional). The client secret is not required if you do not want to let users save and re-use payment methods.
Setup
To be able to use the Dynamic Checkout utilities from our Web SDK, you need to attach the ProcessOut.js script to your HTML file and create a HTML element that will act as the mounting point for Dynamic Checkout:
<div id="dynamic-checkout-container"></div>
<script src="https://js.processout.com/processout.js"></script>
After linking the script your code will have access to the global ProcessOut
instance. This will help you to set up ProcessOut Client using the invoice ID, project ID, and optionally the client secret:
const client = new ProcessOut.ProcessOut('{projectId}');
const dynamicCheckout = client.setupDynamicCheckout({
invoiceId: '{invoiceId}',
projectId: '{projectId}',
clientSecret: '{clientSecret}' // Optional.
});
dynamicCheckout.mount("#dynamic-checkout-container");
After that you should see the Dynamic Checkout widget displayed on your website:
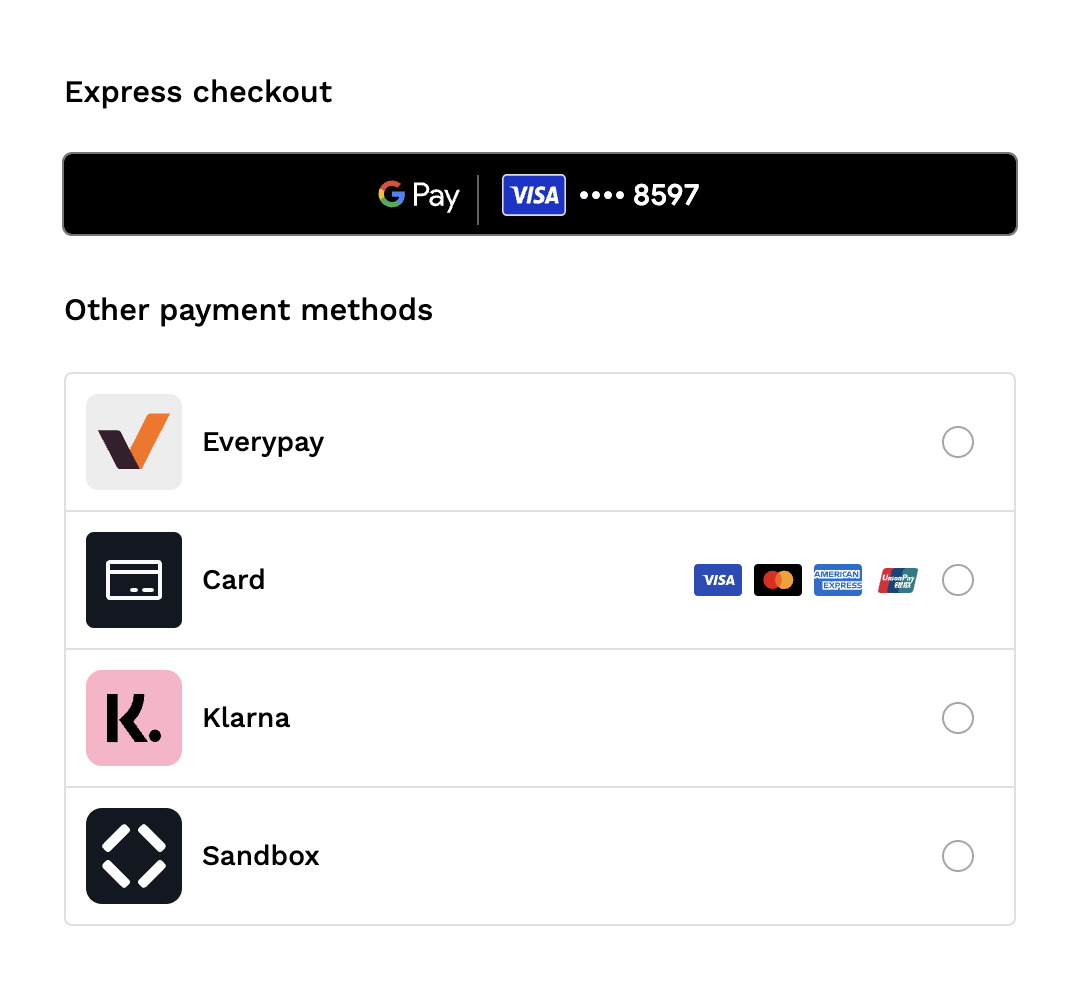
Simple Dynamic Checkout implementation
Reacting to payment flow events
Dynamic Checkout dispatches browser events on every important step of the payment. This enables you to react on them however you like. To react on events you need to add event listeners—for example:
window.addEventListener("processout_dynamic_checkout_payment_success", function (event) {
// Handle the event.
});
Every event has information about it attached. You can see it by accessing event.detail
property.
Event | Description |
---|---|
processout_dynamic_checkout_loading | Widget is loading |
processout_dynamic_checkout_ready | Widget is loaded and ready to perform the payment flow |
processout_dynamic_checkout_invoice_fetching_error | Error when fetching the invoice details |
processout_dynamic_checkout_tokenize_payment_success | Card successfully tokenized |
processout_dynamic_checkout_tokenize_payment_error | Card tokenization error |
processout_dynamic_checkout_payment_error | Payment error |
processout_dynamic_checkout_payment_success | Payment success |
Localization
You can localize all of the text displayed in Dynamic Checkout widget by passing the locale
property when setting up Dynamic Checkout:
const dynamicCheckout = client.setupDynamicCheckout({
invoiceId: '{invoiceId}',
projectId: '{projectId}',
clientSecret: '{clientSecret}',
locale: 'pl'
});
The currently supported locales are en
, es
, fr
, pt
, pl
. Please get in touch with your ProcessOut account manager to request support for new locales.
Customization
You can configure the theme of the widget by passing additional arguments when setting up Dynamic Checkout:
const dynamicCheckout = client.setupDynamicCheckout({
invoiceId: '{invoiceId}',
projectId: '{projectId}',
clientSecret: '{clientSecret}'
}, {
payButtonColor: "red",
payButtonTextColor: "white"
});
Supported theme properties:
Property | Description | Format |
---|---|---|
payButtonColor | Background color of the submit button | CSS color |
payButtonTextColor | Text color of the submit button | CSS color |
Updated 3 months ago
After the payment is successfully authorized you can proceed to capture the payment on your server.